{
"cells": [
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "notes"
}
},
"source": [
"Slide conversion: https://echorand.me/presentation-slides-with-jupyter-notebook.html\n",
"\n",
"`$ jupyter-nbconvert --to slides slides.ipynb --reveal-prefix=reveal.js --post serve`"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"# A Brief Python Introduction\n",
"Keith Maull
\n",
"Jan. 03, 2019"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"## Python is a general purpose programming language that is ...\n",
"\n",
"* interpreted\n",
"* dynamically typed (not statically typed)\n",
"* intuitive\n",
"* fun and addictive"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "notes"
}
},
"source": [
"### strongly typed\n",
"* So to answer your question: another way to look at this that's mostly correct is to say that static typing is compile-time type safety and strong typing is runtime type safety.\n",
"### everything is an object"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"## Python syntax can be learned very quickly\n",
"\n",
"* indentation matters\n",
"* there are no curly braces, semicolons or tricks ...\n",
"* the syntax is one of the true joys of the language once you learn not to fight it\n"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"source": [
"## We will learn 80% of the syntax today in 1 hour!\n",
"\n",
"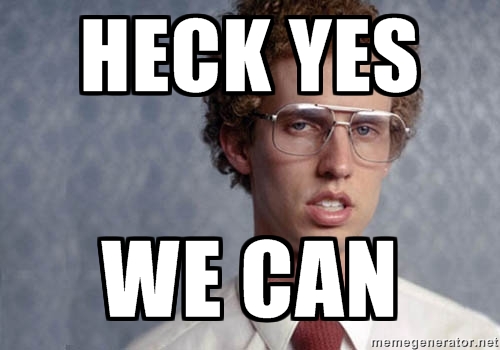"
]
},
{
"cell_type": "code",
"execution_count": 1,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"The first item of the list is an `a`\n"
]
}
],
"source": [
"a_simple_list = ['a', 'b', 'c', 'd']\n",
"if a_simple_list[0] == 'a':\n",
" print(\"The first item of the list is an `a`\")"
]
},
{
"cell_type": "code",
"execution_count": 2,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"a\n",
"b\n",
"c\n",
"d\n"
]
}
],
"source": [
"for item in a_simple_list:\n",
" print(item)"
]
},
{
"cell_type": "code",
"execution_count": 3,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"0\n",
"1\n",
"2\n",
"3\n",
"4\n"
]
}
],
"source": [
"count = 0\n",
"while (count < 5):\n",
" print(count)\n",
" count+=1"
]
},
{
"cell_type": "code",
"execution_count": 4,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"data": {
"text/plain": [
"12"
]
},
"execution_count": 4,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"# functions are easy \n",
"def my_func(x, y):\n",
" return x*y\n",
"\n",
"my_func(3,4)"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "notes"
}
},
"source": [
"### x, y = y, x"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"## Python has many modules (aka \"libraries\") that do fun things"
]
},
{
"cell_type": "code",
"execution_count": 5,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"89\n"
]
}
],
"source": [
"# random numbers generator\n",
"import random\n",
"print(random.randint(0,100))"
]
},
{
"cell_type": "code",
"execution_count": 6,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"aa\n",
"aah\n",
"aahed\n",
"aahing\n",
"aahs\n",
"aal\n",
"aalii\n",
"aaliis\n",
"aals\n",
"aardvark\n",
"aardvarks\n",
"aardwolf\n",
"aardwolves\n",
"aargh\n",
"aarrgh\n",
"aarrghh\n",
"aarti\n",
"aartis\n",
"aas\n",
"aasvogel\n",
"aasvogels\n",
"ab\n",
"aba\n",
"abac\n",
"abaca\n",
"abacas\n",
"abaci\n",
"aback\n",
"abacs\n",
"abacterial\n",
"abactinal\n",
"abactinally\n",
"abactor\n",
"abactors\n",
"abacus\n",
"abacuses\n",
"abaft\n",
"abaka\n",
"abakas\n",
"abalone\n",
"abalones\n",
"abamp\n",
"abampere\n",
"abamperes\n",
"abamps\n",
"aband\n",
"abanded\n",
"abanding\n",
"abandon\n",
"abandoned\n",
"abandonedly\n",
"abandonee\n",
"abandonees\n",
"abandoner\n",
"abandoners\n",
"abandoning\n",
"abandonment\n",
"abandonments\n",
"abandons\n",
"abandonware\n",
"abandonwares\n",
"abands\n",
"abapical\n",
"abas\n",
"abase\n",
"abased\n",
"abasedly\n",
"abasement\n",
"abasements\n",
"abaser\n",
"abasers\n",
"abases\n",
"abash\n",
"abashed\n",
"abashedly\n",
"abashes\n",
"abashing\n",
"abashless\n",
"abashment\n",
"abashments\n",
"abasia\n",
"abasias\n",
"abasing\n",
"abask\n",
"abatable\n",
"abate\n",
"abated\n",
"abatement\n",
"abatements\n",
"abater\n",
"abaters\n",
"abates\n",
"abating\n",
"abatis\n",
"abatises\n",
"abator\n",
"abators\n",
"abattis\n",
"abattises\n",
"abattoir\n",
"abattoirs\n",
"abattu\n",
"abature\n",
"abatures\n",
"abaxial\n",
"abaxile\n",
"abaya\n",
"abayas\n",
"abb\n",
"abba\n",
"abbacies\n",
"abbacy\n",
"abbas\n",
"abbatial\n",
"abbe\n",
"abbed\n",
"abbes\n",
"abbess\n",
"abbesses\n",
"abbey\n",
"abbeys\n",
"abbot\n",
"abbotcies\n",
"abbotcy\n",
"abbots\n",
"abbotship\n",
"abbotsh\n"
]
}
],
"source": [
"# http library\n",
"import requests\n",
"r = requests.get(\"https://raw.githubusercontent.com/atebits/Words/master/Words/en.txt\")\n",
"if r.status_code == 200:\n",
" data = r.text\n",
" print(data[:1000])"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"source": [
"## And a strength of the language is text processing ..."
]
},
{
"cell_type": "code",
"execution_count": 7,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"['aa',\n",
" 'aah',\n",
" 'aahed',\n",
" 'aahing',\n",
" 'aahs',\n",
" 'aal',\n",
" 'aalii',\n",
" 'aaliis',\n",
" 'aals',\n",
" 'aardvark']"
]
},
"execution_count": 7,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"data.split('\\n')[:10]"
]
},
{
"cell_type": "code",
"execution_count": 8,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"['aahed', 'aalii', 'aargh', 'aarti', 'abaca', 'abaci', 'aback', 'abacs', 'abaft', 'abaka', 'abamp', 'aband', 'abase', 'abash', 'abask', 'abate', 'abaya', 'abbas', 'abbed', 'abbes', 'abbey', 'abbot', 'abcee', 'abeam', 'abear', 'abele', 'abets', 'abhor', 'abide', 'abies', 'abled', 'abler', 'ables', 'ablet', 'ablow', 'abmho', 'abode', 'abohm', 'aboil', 'aboma', 'aboon', 'abord', 'abore', 'abort', 'about', 'above', 'abram', 'abray', 'abrim', 'abrin', 'abris', 'absey', 'absit', 'abuna', 'abune', 'abuse', 'abuts', 'abuzz', 'abyes', 'abysm', 'abyss', 'acais', 'acari', 'accas', 'accoy', 'acerb', 'acers', 'aceta', 'achar', 'ached', 'aches', 'achoo', 'acids', 'acidy', 'acing', 'acini', 'ackee', 'acker', 'acmes', 'acmic', 'acned', 'acnes', 'acock', 'acold', 'acorn', 'acred', 'acres', 'acrid', 'acted', 'actin', 'acton', 'actor', 'acute', 'acyls', 'adage', 'adapt', 'adaws', 'adays', 'addax', 'added']\n"
]
}
],
"source": [
"top_100_five_letter_words = []\n",
"\n",
"for w in data.split('\\n'):\n",
" if len(w) == 5:\n",
" top_100_five_letter_words.append(w)\n",
" if len(top_100_five_letter_words) == 100:\n",
" break\n",
"\n",
"print(top_100_five_letter_words)"
]
},
{
"cell_type": "code",
"execution_count": 9,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"['acing', 'aging', 'ahing', 'aking', 'alang', 'almug', 'along', 'among', 'aping', 'awing', 'axing', 'befog', 'being', 'bewig', 'bhang', 'bling', 'boing', 'boong', 'bourg', 'bring', 'brung', 'chang', 'clang', 'cling', 'clung', 'cohog', 'colog', 'craig', 'cuing', 'debag', 'debug', 'defog', 'derig', 'doing', 'droog', 'duing', 'dwang', 'dying', 'ehing', 'eking', 'embog', 'emong', 'ennog', 'ering', 'exing', 'eying', 'fling', 'flong', 'flung', 'glogg', 'going', 'gulag', 'hoing', 'hying', 'hyleg', 'icing', 'incog', 'iring', 'kaing', 'kiang', 'klang', 'klieg', 'klong', 'krang', 'kreng', 'kyang', 'liang', 'lolog', 'lying', 'moong', 'nying', 'obang', 'oflag', 'ohing', 'oping', 'orang', 'owing', 'phang', 'piing', 'pirog', 'pling', 'plong', 'prang', 'prong', 'rejig', 'renig', 'repeg', 'rerig', 'retag', 'rolag', 'ruing', 'scoog', 'scoug', 'scrag', 'scrog', 'shrug', 'skegg', 'slang', 'sling', 'slung']\n"
]
}
],
"source": [
"top_100_five_letter_words_ending_in_g = []\n",
"\n",
"for w in data.split('\\n'):\n",
" if len(w) == 5 and w[-1] is 'g':\n",
" top_100_five_letter_words_ending_in_g.append(w)\n",
" if len(top_100_five_letter_words_ending_in_g) == 100:\n",
" break\n",
"\n",
"print(top_100_five_letter_words_ending_in_g)"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"### json support is excellent (as it should be)"
]
},
{
"cell_type": "code",
"execution_count": 10,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"name": "stderr",
"output_type": "stream",
"text": [
"IOPub data rate exceeded.\n",
"The notebook server will temporarily stop sending output\n",
"to the client in order to avoid crashing it.\n",
"To change this limit, set the config variable\n",
"`--NotebookApp.iopub_data_rate_limit`.\n",
"\n",
"Current values:\n",
"NotebookApp.iopub_data_rate_limit=1000000.0 (bytes/sec)\n",
"NotebookApp.rate_limit_window=3.0 (secs)\n",
"\n"
]
}
],
"source": [
"import json\n",
"import requests\n",
"\n",
"r = requests.get(\"https://raw.githubusercontent.com/LearnWebCode/json-example/master/pets-data.json\")\n",
"if r.status_code == 200:\n",
" pet_data = json.loads(r.text)\n",
"print(data)"
]
},
{
"cell_type": "code",
"execution_count": 11,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"{'name': 'Purrsloud',\n",
" 'species': 'Cat',\n",
" 'favFoods': ['wet food', 'dry food', 'any food'],\n",
" 'birthYear': 2016,\n",
" 'photo': 'https://learnwebcode.github.io/json-example/images/cat-2.jpg'}"
]
},
"execution_count": 11,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"pet_data['pets'][0]"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"source": [
"### Regular expressions ... also very accessible"
]
},
{
"cell_type": "code",
"execution_count": 12,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"tabasheers\n",
"tabers\n",
"tabliers\n",
"taborers\n",
"tabourers\n",
"tacheometers\n",
"tachometers\n",
"tachygraphers\n",
"tachymeters\n",
"tackers\n",
"tackifiers\n",
"tacklers\n",
"taggers\n",
"tailenders\n",
"tailers\n",
"tailgaters\n",
"tailwaters\n",
"taivers\n",
"takeovers\n",
"takers\n",
"talebearers\n",
"talers\n",
"talkers\n",
"talliers\n",
"tambers\n",
"tambourers\n",
"tamers\n",
"tamperers\n",
"tampers\n",
"tanagers\n",
"tanglers\n",
"tankbusters\n",
"tankers\n",
"tanners\n",
"tantalisers\n",
"tantalizers\n",
"taperers\n",
"tapers\n",
"tappers\n",
"tapsters\n",
"targeteers\n",
"tarnishers\n",
"tarpapers\n",
"tarriers\n",
"tarsiers\n",
"taseometers\n",
"tasers\n",
"tasimeters\n",
"taskers\n",
"taskmasters\n",
"tastemakers\n",
"tasters\n",
"taters\n",
"tatlers\n",
"tatters\n",
"tattlers\n",
"tattooers\n",
"taunters\n",
"tautomers\n",
"taverners\n",
"tavers\n",
"tawers\n",
"taxameters\n",
"taxers\n",
"taximeters\n",
"taxonomers\n",
"taxpayers\n",
"teachers\n",
"teamakers\n",
"teamers\n",
"teamsters\n",
"tearers\n",
"tearjerkers\n",
"teaselers\n",
"teasellers\n",
"teasers\n",
"teatasters\n",
"tedders\n",
"teemers\n",
"teenagers\n",
"teeners\n",
"teenyboppers\n",
"teers\n",
"teeters\n",
"teethers\n",
"teetotalers\n",
"teetotallers\n",
"telecasters\n",
"telecommuters\n",
"teleconverters\n",
"telegraphers\n",
"telemarketers\n",
"telemeters\n",
"telephoners\n",
"teleprinters\n",
"teletypewriters\n",
"televiewers\n",
"televisers\n",
"teleworkers\n",
"telewriters\n",
"telfers\n",
"tellers\n",
"tellurometers\n",
"telphers\n",
"temperers\n",
"tempers\n",
"temporisers\n",
"temporizers\n",
"tempters\n",
"tenderers\n",
"tenderisers\n",
"tenderizers\n",
"tenderometers\n",
"tenders\n",
"tenners\n",
"tenoners\n",
"tenpounders\n",
"tensimeters\n",
"tensiometers\n",
"tensioners\n",
"tenters\n",
"tentmakers\n",
"termers\n",
"terminers\n",
"terpolymers\n",
"terriers\n",
"terrifiers\n",
"terrorisers\n",
"terrorizers\n",
"testers\n",
"testifiers\n",
"tethers\n",
"tetramers\n",
"tetrameters\n",
"tetters\n",
"teuchters\n",
"texters\n",
"thalers\n",
"thankers\n",
"thanksgivers\n",
"thatchers\n",
"thawers\n",
"theatergoers\n",
"theaters\n",
"theologasters\n",
"theologers\n",
"theologisers\n",
"theologizers\n",
"theorisers\n",
"theorizers\n",
"theosophers\n",
"thermographers\n",
"thermometers\n",
"thickeners\n",
"thiggers\n",
"thillers\n",
"thimbleriggers\n",
"thinkers\n",
"thinners\n",
"thirsters\n",
"thrashers\n",
"threaders\n",
"threadmakers\n",
"threapers\n",
"threateners\n",
"threepers\n",
"threshers\n",
"thrillers\n",
"thrivers\n",
"throbbers\n",
"thronners\n",
"throttlers\n",
"throwers\n",
"throwsters\n",
"thrummers\n",
"thrusters\n",
"thumpers\n",
"thunderers\n",
"thunders\n",
"thundershowers\n",
"thurifers\n",
"thwackers\n",
"thwarters\n",
"tickers\n",
"ticklers\n",
"tiddlers\n",
"tidewaiters\n",
"tidewaters\n",
"tidiers\n",
"tiebreakers\n",
"tiers\n",
"tigers\n",
"tighteners\n",
"tilers\n",
"tillers\n",
"tilters\n",
"tiltmeters\n",
"timbers\n",
"timekeepers\n",
"timepleasers\n",
"timers\n",
"timesavers\n",
"timeservers\n",
"timeworkers\n",
"timoneers\n",
"tinders\n",
"tinglers\n",
"tinkerers\n",
"tinkers\n",
"tinklers\n",
"tinners\n",
"tinters\n",
"tintometers\n",
"tippers\n",
"tipplers\n",
"tipsters\n",
"titers\n",
"titfers\n",
"tithers\n",
"titleholders\n",
"titlers\n",
"titterers\n",
"titters\n",
"toadeaters\n",
"toasters\n",
"toastmasters\n",
"tobogganers\n",
"tochers\n",
"toddlers\n",
"todgers\n",
"toeraggers\n",
"toggers\n",
"togglers\n",
"toilers\n",
"tokers\n",
"tollers\n",
"tolters\n",
"toners\n",
"tongers\n",
"tongsters\n",
"tonguesters\n",
"tonkers\n",
"tonners\n",
"tonometers\n",
"tontiners\n",
"toolers\n",
"toolholders\n",
"toolmakers\n",
"toolpushers\n",
"tooters\n",
"tootlers\n",
"topers\n",
"topliners\n",
"topmakers\n",
"topnotchers\n",
"topographers\n",
"toppers\n",
"topsiders\n",
"torchbearers\n",
"torchers\n",
"torchiers\n",
"tormenters\n",
"torpedoers\n",
"torquers\n",
"torturers\n",
"toshers\n",
"tossers\n",
"totalisers\n",
"totalizers\n",
"toters\n",
"totterers\n",
"totters\n",
"touchers\n",
"touchpapers\n",
"tougheners\n",
"tourers\n",
"tourneyers\n",
"tousers\n",
"touters\n",
"towers\n",
"towsers\n",
"toyers\n",
"tracers\n",
"trackers\n",
"tracklayers\n",
"trackwalkers\n",
"traders\n",
"traditioners\n",
"traducers\n",
"traffickers\n",
"trailblazers\n",
"trailbreakers\n",
"trailers\n",
"trainbearers\n",
"trainers\n",
"trammelers\n",
"trammellers\n",
"trampers\n",
"tramplers\n",
"trampoliners\n",
"tranquilisers\n",
"tranquilizers\n",
"tranquillisers\n",
"tranquillizers\n",
"transceivers\n",
"transcribers\n",
"transducers\n",
"transferrers\n",
"transfers\n",
"transformers\n",
"transfusers\n",
"transgenders\n",
"transhippers\n",
"transmissometers\n",
"transmitters\n",
"transmuters\n",
"transplanters\n",
"transponders\n",
"transporters\n",
"transposers\n",
"transputers\n",
"transshippers\n",
"transvaluers\n",
"transverters\n",
"tranters\n",
"trapanners\n",
"trappers\n",
"trapshooters\n",
"trashers\n",
"travelers\n",
"travellers\n",
"traversers\n",
"trawlers\n",
"treacherers\n",
"treachers\n",
"treaders\n",
"treadlers\n",
"treasurers\n",
"treaters\n",
"treehoppers\n",
"trekkers\n",
"tremblers\n",
"trenchers\n",
"trendsetters\n",
"trepanners\n",
"trephiners\n",
"trespassers\n",
"triaconters\n",
"tribometers\n",
"tributers\n",
"trickers\n",
"tricksters\n",
"tricyclers\n",
"triers\n",
"triflers\n",
"triggers\n",
"trigonometers\n",
"trillers\n",
"trimers\n",
"trimesters\n",
"trimeters\n",
"trimmers\n",
"trinketers\n",
"triphammers\n",
"trippers\n",
"tripplers\n",
"triumphers\n",
"trochanters\n",
"trocheameters\n",
"trochometers\n",
"troffers\n",
"trollers\n",
"tromometers\n",
"troopers\n",
"troposcatters\n",
"trossers\n",
"trotters\n",
"troublemakers\n",
"troublers\n",
"troubleshooters\n",
"trouncers\n",
"troupers\n",
"trousers\n",
"trouters\n",
"trovers\n",
"trowelers\n",
"trowellers\n",
"trowsers\n",
"truckers\n",
"trucklers\n",
"truckmasters\n",
"trudgers\n",
"trumpeters\n",
"truncheoners\n",
"trundlers\n",
"trussers\n",
"trustbusters\n",
"trusters\n",
"tryers\n",
"trysters\n",
"tubbers\n",
"tubers\n",
"tuckers\n",
"tufters\n",
"tuggers\n",
"tumblers\n",
"tummlers\n",
"tuners\n",
"tunnelers\n",
"tunnellers\n",
"turbidimeters\n",
"turbochargers\n",
"turcopoliers\n",
"turners\n",
"turnovers\n",
"turtlers\n",
"tushkers\n",
"tuskers\n",
"tussers\n",
"tutoyers\n",
"tutworkers\n",
"tuyers\n",
"twaddlers\n",
"twangers\n",
"twanglers\n",
"twattlers\n",
"tweakers\n",
"tweedlers\n",
"tweenagers\n",
"tweeners\n",
"tweers\n",
"tweeters\n",
"tweezers\n",
"twicers\n",
"twiddlers\n",
"twiers\n",
"twiggers\n",
"twiners\n",
"twinflowers\n",
"twinklers\n",
"twinters\n",
"twirlers\n",
"twisters\n",
"twitchers\n",
"twitterers\n",
"twitters\n",
"twoccers\n",
"twockers\n",
"twoers\n",
"twofers\n",
"twoseaters\n",
"twyers\n",
"tyers\n",
"tylers\n",
"typecasters\n",
"typefounders\n",
"typesetters\n",
"typewriters\n",
"typifiers\n",
"typographers\n",
"tyrannisers\n",
"tyrannizers\n"
]
}
],
"source": [
"# regular expressions\n",
"import re\n",
"s = r'^t.*ers$' # all words starting with `t` ending in `ers`\n",
"\n",
"for w in data.split('\\n'):\n",
" if re.match(s, w):\n",
" print(w)"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"source": [
"### There are many thousands of libraries to get lost in ..."
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"## Data types in Python are simple and complete ...\n",
"\n",
"* You are already familiar with these:\n",
" * numbers (`1`, `1.87`, `-0.88`, ...)\n",
" * strings (`\"Hello\"`, `'Hello'`)\n",
" * Boolean (`True`, `False`)\n",
" * `None`"
]
},
{
"cell_type": "code",
"execution_count": 13,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [],
"source": [
"### No surprises here ..."
]
},
{
"cell_type": "code",
"execution_count": 14,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"3"
]
},
"execution_count": 14,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"1 + 2"
]
},
{
"cell_type": "code",
"execution_count": 15,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"'helloHello'"
]
},
"execution_count": 15,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"'hello' + \"Hello\""
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"## Iterables are an important type category that includes:\n",
"\n",
"* lists\n",
"* tuples\n",
"* sets\n"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"source": [
"### Lists are just like arrays ..."
]
},
{
"cell_type": "code",
"execution_count": 16,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"2\n"
]
}
],
"source": [
"lst = [1, 2, \"three\"]\n",
"print(lst[1])"
]
},
{
"cell_type": "code",
"execution_count": 17,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"1 2 three "
]
}
],
"source": [
"for i in lst:\n",
" print(i, end=\" \")"
]
},
{
"cell_type": "code",
"execution_count": 18,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"[1, 2, 'three', 'a', 'b']"
]
},
"execution_count": 18,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"lst + ['a', 'b']"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"source": [
"### Python doesn't require you to keep up with indices ...\n",
"\n",
"* but if you need them (which you rarely will) use `enumerate`"
]
},
{
"cell_type": "code",
"execution_count": 19,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"0:1 1:2 2:three "
]
}
],
"source": [
"for idx, l in enumerate(lst):\n",
" print(\"{}:{}\".format(idx, l), end=\" \")"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"source": [
"### You can also do cool things with list access ..."
]
},
{
"cell_type": "code",
"execution_count": 20,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"'three'"
]
},
"execution_count": 20,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"lst[-1]"
]
},
{
"cell_type": "code",
"execution_count": 21,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"[1, 2]"
]
},
"execution_count": 21,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"lst[0:2]"
]
},
{
"cell_type": "code",
"execution_count": 22,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"['three', 2, 1]"
]
},
"execution_count": 22,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"lst[::-1]"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"source": [
"### Tuples are another useful type\n",
"* they are just immutable lists\n",
"* and denoted `(1, 2, 'three`)"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"source": [
"### Sets are also valuable "
]
},
{
"cell_type": "code",
"execution_count": 23,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"{1, 2, 3, 4, 5}"
]
},
"execution_count": 23,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"set([1,1,1,1,1,1,2,3,4,5,5,5,5,5,5,5])"
]
},
{
"cell_type": "code",
"execution_count": 24,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"{1, 2, 3, 4, 5}"
]
},
"execution_count": 24,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"setA = set([1,2])\n",
"setB = set([1,2,3,4,5])\n",
"setA.union(setB)"
]
},
{
"cell_type": "code",
"execution_count": 25,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"set()"
]
},
"execution_count": 25,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"setA.difference(setB)"
]
},
{
"cell_type": "code",
"execution_count": 26,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"{3, 4, 5}"
]
},
"execution_count": 26,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"setB.difference(setA)"
]
},
{
"cell_type": "code",
"execution_count": 27,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"{1, 2}"
]
},
"execution_count": 27,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"setA.intersection(setB)"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"## Dictionaries are also a crucial type in the language\n",
"\n",
"* associative arrays\n",
"* key/value pairs"
]
},
{
"cell_type": "code",
"execution_count": 28,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"{'a': 5, 'b': 6}\n"
]
}
],
"source": [
"d = {\"a\": 5, \"b\": 6}\n",
"print(d)"
]
},
{
"cell_type": "code",
"execution_count": 29,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"{'a': 5, 'b': 6}\n"
]
}
],
"source": [
"d = dict([('a', 5), ('b', 6)])\n",
"print(d)"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"### Providing the usual suspects ..."
]
},
{
"cell_type": "code",
"execution_count": 30,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"5"
]
},
"execution_count": 30,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"d['a']"
]
},
{
"cell_type": "code",
"execution_count": 31,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"dict_keys(['a', 'b'])"
]
},
"execution_count": 31,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"d.keys()"
]
},
{
"cell_type": "code",
"execution_count": 32,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"dict_values([5, 6])"
]
},
"execution_count": 32,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"d.values()"
]
},
{
"cell_type": "code",
"execution_count": 33,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"a=>5\n",
"b=>6\n"
]
}
],
"source": [
"for key, value in d.items():\n",
" print(\"{}=>{}\".format(key, value))"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"## Python logic operators are intuitive\n",
"\n",
"* you've seen `in` and `is`\n",
"* but there is `not`, `not in`, `is not`, `and`, `or`"
]
},
{
"cell_type": "code",
"execution_count": 34,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"data": {
"text/plain": [
"False"
]
},
"execution_count": 34,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"1 is 2"
]
},
{
"cell_type": "code",
"execution_count": 35,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"True"
]
},
"execution_count": 35,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"1 is not 2"
]
},
{
"cell_type": "code",
"execution_count": 36,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"True"
]
},
"execution_count": 36,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"True and True"
]
},
{
"cell_type": "code",
"execution_count": 37,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"True"
]
},
"execution_count": 37,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"True or False"
]
},
{
"cell_type": "code",
"execution_count": 38,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"data": {
"text/plain": [
"False"
]
},
"execution_count": 38,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"False or False"
]
},
{
"cell_type": "code",
"execution_count": 39,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"True"
]
},
"execution_count": 39,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"False is not True"
]
},
{
"cell_type": "code",
"execution_count": 40,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [],
"source": [
"s = 'supercalifragilistic'"
]
},
{
"cell_type": "code",
"execution_count": 41,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"True"
]
},
"execution_count": 41,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"'u' in s"
]
},
{
"cell_type": "code",
"execution_count": 42,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"True"
]
},
"execution_count": 42,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"'z' not in s"
]
},
{
"cell_type": "code",
"execution_count": 43,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [],
"source": [
"lst = ['a', 'b', 1, 2, 3]"
]
},
{
"cell_type": "code",
"execution_count": 44,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"True"
]
},
"execution_count": 44,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"'a' in lst"
]
},
{
"cell_type": "code",
"execution_count": 45,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"data": {
"text/plain": [
"True"
]
},
"execution_count": 45,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"s == 'supercalifragilistic'"
]
},
{
"cell_type": "code",
"execution_count": 46,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"True"
]
},
"execution_count": 46,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"s is 'supercalifragilistic'"
]
},
{
"cell_type": "code",
"execution_count": 47,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"True"
]
},
"execution_count": 47,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"lst == ['a', 'b', 1, 2, 3]"
]
},
{
"cell_type": "code",
"execution_count": 48,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"False"
]
},
"execution_count": 48,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"lst is ['a', 'b', 1, 2, 3]"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"## Flow control in Python also includes all the usuals ...\n",
"* `if/elif/else`\n",
"* `for`\n",
"* `while`"
]
},
{
"cell_type": "code",
"execution_count": 49,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"the first letter is a 't'\n"
]
}
],
"source": [
"s = 'this is a test'\n",
"\n",
"if s[0] == 't':\n",
" print(\"the first letter is a 't'\")\n",
"else:\n",
" print(\"the first letter is not a 't'\")"
]
},
{
"cell_type": "code",
"execution_count": 50,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"t\n",
"h\n",
"i\n",
"s\n",
" \n",
"i\n",
"s\n",
" \n",
"a\n",
" \n",
"t\n",
"e\n",
"s\n",
"t\n"
]
}
],
"source": [
"for c in s:\n",
" print(\"{}\".format(c))"
]
},
{
"cell_type": "code",
"execution_count": 51,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"t\n",
"h\n",
"i\n",
"s\n",
" \n",
"i\n",
"s\n",
" \n",
"a\n",
" \n",
"t\n",
"e\n",
"s\n",
"t\n"
]
}
],
"source": [
"# THIS IS NOT PYTHONIC! for is more elegant\n",
"\n",
"i = 0\n",
"while(i < len(s)):\n",
" print(\"{}\".format(s[i]))\n",
" i+=1"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "skip"
}
},
"source": [
"## classes/objects"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"## Python's built in functions are excellent\n",
"* There are about 69 functions\n",
"* some of the most common/useful are:\n",
" * `min`, `max`, `all`, `any` \n",
" * `zip`, `range`, `len`, `map`, `sorted`\n",
" * `reversed`"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"### Let's play:\n",
"\n",
"* **PROBLEM**: create a 16 character random password of upper, lower and number\n",
"* **ONE SOLUTION**: _use what we know about the ASCII table and the `chr()` built in function_"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"source": [
"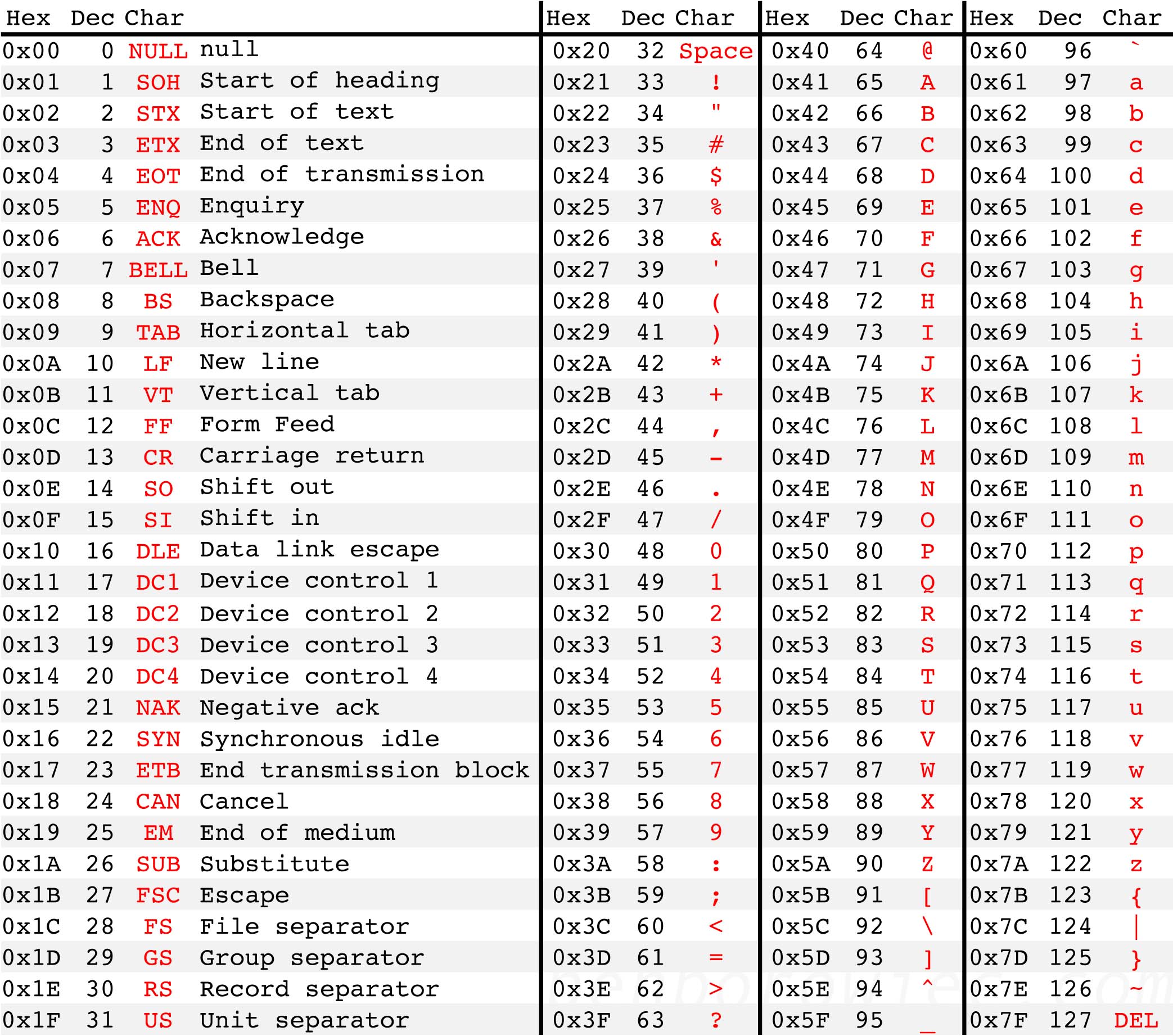"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"source": [
"**HINT 1:**\n",
" "
]
},
{
"cell_type": "code",
"execution_count": 52,
"metadata": {
"slideshow": {
"slide_type": "-"
}
},
"outputs": [
{
"data": {
"text/plain": [
"'z'"
]
},
"execution_count": 52,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"chr(122)"
]
},
{
"cell_type": "code",
"execution_count": 53,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"uMVKgvYyB114e4rpj"
]
}
],
"source": [
"import random\n",
"\n",
"for i in range(0, 17):\n",
" # get a number in the ASCII range\n",
" while(True):\n",
" rnd_c = random.randint(48, 123)\n",
" if rnd_c < 58 or (rnd_c > 65 and rnd_c <91) or (rnd_c > 96 and rnd_c < 123):\n",
" break\n",
" \n",
" print(chr(rnd_c), end=\"\")"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"## Functions are straightforward and intuitive"
]
},
{
"cell_type": "code",
"execution_count": 54,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"1"
]
},
"execution_count": 54,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"def a_function():\n",
" return 1\n",
"\n",
"a_function()"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"source": [
"### Function arguments (parameters) come in three flavors\n",
"\n",
"* positional\n",
"* keyword\n",
"* mixed\n",
"\n",
"Note: default values are allowed!"
]
},
{
"cell_type": "code",
"execution_count": 55,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"data": {
"text/plain": [
"6"
]
},
"execution_count": 55,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"def a_function(a, b, c):\n",
" return a*b*c\n",
"\n",
"a_function(1,2,3)"
]
},
{
"cell_type": "code",
"execution_count": 56,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"data": {
"text/plain": [
"192"
]
},
"execution_count": 56,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"def a_function(a=1, b=1, c=1):\n",
" return a*b*c\n",
"\n",
"a_function(a=3,b=8,c=8)"
]
},
{
"cell_type": "code",
"execution_count": 57,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"4"
]
},
"execution_count": 57,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"a_function(a=4)"
]
},
{
"cell_type": "code",
"execution_count": 58,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"48"
]
},
"execution_count": 58,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"a_function(3,b=4,c=4)"
]
},
{
"cell_type": "code",
"execution_count": 59,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"data": {
"text/plain": [
"384"
]
},
"execution_count": 59,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"def a_function(a, b=1, c=1):\n",
" return a*b*c\n",
"\n",
"a_function(6,b=8,c=8)"
]
},
{
"cell_type": "code",
"execution_count": 60,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"384"
]
},
"execution_count": 60,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"a_function(6, 8, 8)"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"source": [
"### Functions can return multiple values with tuples"
]
},
{
"cell_type": "code",
"execution_count": 61,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [],
"source": [
"def b_function():\n",
" return (1, 2, 3)"
]
},
{
"cell_type": "code",
"execution_count": 62,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"(1, 2, 3)"
]
},
"execution_count": 62,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"b_function()"
]
},
{
"cell_type": "code",
"execution_count": 63,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"1 2 3\n"
]
}
],
"source": [
"x, y, z = b_function()\n",
"print(x, y, z)"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"## Exceptions are valuable for good code!\n",
"* Python supports `try`/`except`/`finally` and they should be used regularly"
]
},
{
"cell_type": "code",
"execution_count": 64,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [],
"source": [
"def c_function(a, b, c):\n",
" try:\n",
" if a<0:\n",
" raise Exception\n",
" return a*b*c\n",
" except Exception as e:\n",
" print(\"The first parameter cannot be less than zero.\")\n",
" finally:\n",
" pass # we don't need to clean up anything after the exception"
]
},
{
"cell_type": "code",
"execution_count": 65,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"The first parameter cannot be less than zero.\n"
]
}
],
"source": [
"c_function(-1, 2, 3) "
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"source": [
"### What did the function _return_ from the exception???"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"source": [
"ALL PYTHON FUNCTIONS IMPLICITLY RETURN `None` WHEN NO RETURN VALUE IS SPECIFIED"
]
},
{
"cell_type": "code",
"execution_count": 66,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"The first parameter cannot be less than zero.\n"
]
},
{
"data": {
"text/plain": [
"True"
]
},
"execution_count": 66,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"c_function(-1, 2, 3) is None"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"## Comprehensions"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"source": [
"### List comprehensions provide shorthand for building lists"
]
},
{
"cell_type": "code",
"execution_count": 67,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]"
]
},
"execution_count": 67,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"l1 = [x for x in range(0,11)]\n",
"l1"
]
},
{
"cell_type": "code",
"execution_count": 68,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"data": {
"text/plain": [
"'TMbD0xr0Bj'"
]
},
"execution_count": 68,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"def pw_generator():\n",
" ascii_pw_range = list(range(48,58)) + list(range(65,91)) + list(range (97, 123))\n",
" \n",
" return ''.join([chr(random.choice(ascii_pw_range)) \\\n",
" for i in range(0,10)])\n",
"\n",
"pw_generator()"
]
},
{
"cell_type": "code",
"execution_count": 69,
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"outputs": [
{
"data": {
"text/plain": [
"['MIO3ewedYc',\n",
" 'YbFp3ofMhC',\n",
" 'lgy5GUvod4',\n",
" 'VfOjbJ1oF3',\n",
" 'tqYltK37KK',\n",
" 'E4SzZ0zRjX',\n",
" 'fdJRpvyHrn',\n",
" '9cCas9FpyA',\n",
" '33qhlSo7kd',\n",
" 'dL1QupAp0V',\n",
" 'KBciH7ZSaN',\n",
" '3B1StGRLLe',\n",
" 'bR6wZCAzsa',\n",
" '9gq3zcB6lb',\n",
" 'vmNodUhosK']"
]
},
"execution_count": 69,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"[pw_generator() for i in range(0,15)]"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "subslide"
}
},
"source": [
"### Dictionary comprehensions are also quite nice ...\n",
"\n",
"* Let's say you want to swap the key, value pairs in a dictionary ..."
]
},
{
"cell_type": "code",
"execution_count": 70,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"{1: 'a', 2: 'b', 3: 'c'}"
]
},
"execution_count": 70,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"d1 = dict([('a', 1), ('b', 2), ('c', 3)])\n",
"{ v:k for (k,v) in d1.items() }"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"source": [
"* something more practical might be to filter out all values not meeting a criterion"
]
},
{
"cell_type": "code",
"execution_count": 71,
"metadata": {
"slideshow": {
"slide_type": "fragment"
}
},
"outputs": [
{
"data": {
"text/plain": [
"{1: 'a', 3: 'c'}"
]
},
"execution_count": 71,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"d1 = dict([('a', 1), ('b', 2), ('c', 3)])\n",
"{ v:k for (k,v) in d1.items() if v%2 != 0}"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "slide"
}
},
"source": [
"## LET'S PLAY!"
]
},
{
"cell_type": "markdown",
"metadata": {
"slideshow": {
"slide_type": "skip"
}
},
"source": [
"## file i/o"
]
}
],
"metadata": {
"celltoolbar": "Slideshow",
"kernelspec": {
"display_name": "Python 3",
"language": "python",
"name": "python3"
},
"language_info": {
"codemirror_mode": {
"name": "ipython",
"version": 3
},
"file_extension": ".py",
"mimetype": "text/x-python",
"name": "python",
"nbconvert_exporter": "python",
"pygments_lexer": "ipython3",
"version": "3.6.6"
}
},
"nbformat": 4,
"nbformat_minor": 2
}